If you own a website, you are paying for web hosting every month, but are you getting the most out of it? Most people just have a lot of stagnant pages sitting up in cyberspace and are not making the most of them. This is a list of some things you can do to improve your website in cyberspace. The first thing you need to start doing is keep track of how many hits you are getting to your website. How can you track the effectiveness of website changes if you don't know where your visitors are coming from.
You need to have things for people to do on your website. If you're selling software, you could offer a free e-book on how to use the software, for example. You could have an instructional video for them to watch. If you're a musician in a band or a voice over actor, you could offer one free audio download and keep on adding a new download every month.
You need to have a good linking structure in your website and make sure that there are no broken links. Every page of your website should link to each other. Be careful about what websites you link to also, because you might throw away a sale to a competitor.
Offer to send your visitors a free newsletter. When they sign up, ask for more information than just their e-mail address. Ask them where they live, what they do, and what their interests are. It's really up to you to determine what you should be asking. Just be careful, asking to much information can drive them away. By doing this you will find out valuable information about the people who visit your website and are interested in your business. There are companies that provide newsletter services for you, so no knowledge of programming is required. You just need to sign up with a newsletter service. Their are also companies that will provide shopping cart services for your websites so you don't have to spend time programming this either or paying someone to do it. Shopping carts will not necessarily keep track of who purchased what items, so it might be best to pay to have a programmer design your site so that you can know when someone is a repeat customer. This is the kind of customization Amazon.com has.
You need to provide fresh content daily that is of value to your website visitors. And have sections that are updated regularly, at least twice monthly. People will get bored and not come back to your website if they always see the same content.
A new way to provide fresh daily content is blogging. You can set up as many blogs as you want for free and they are very popular with people who surf the internet. Another popular thing to do is to provide topic specific news updates. People will start to see you as an authority on a certain subject if you provide regular updates online.
Just keep updating your website with fresh content. The search engines will love it, and people will too! Soon you will have repeat customers coming back for more. Start today and do something to spice up your website.
1. Given a rectangular (cuboidal for the puritans) cake with a rectangular piece removed (any size or orientation), how would you cut the remainder of the cake into two equal halves with one straight cut of a knife ?
2. You’re given an array containing both positive and negative integers and required to find the subarray with the largest sum (O(N) a la KBL). Write a routine in C for the above.
3. Given an array of size N in which every number is between 1 and N, determine if there are any duplicates in it. You are allowed to destroy the array if you like. [ I ended up giving about 4 or 5 different solutions for this, each supposedly better than the others ].
4. Write a routine to draw a circle (x ** 2 + y ** 2 = r ** 2) without making use of any floating point computations at all. [ This one had me stuck for quite some time and I first gave a solution that did have floating point computations ].
5. Given only putchar (no sprintf, itoa, etc.) write a routine putlong that prints out an unsigned long in decimal. [ I gave the obvious solution of taking % 10 and / 10, which gives us the decimal value in reverse order. This requires an array since we need to print it out in the correct order. The interviewer wasn’t too pleased and asked me to give a solution which didn’t need the array ].
6. Give a one-line C expression to test whether a number is a power of 2. [No loops allowed - it’s a simple test.]
7. Given an array of characters which form a sentence of words, give an efficient algorithm to reverse the order of the words (not characters) in it.
8. How many points are there on the globe where by walking one mile south, one mile east and one mile north you reach the place where you started.
9. Give a very good method to count the number of ones in a 32 bit number. (caution: looping through testing each bit is not a solution).
10. What are the different ways to say, the value of x can be either a 0 or a 1. Apparently the if then else solution has a jump when written out in assembly. if (x == 0) y=0 else y =x There is a logical, arithmetic and a datastructure soln to the above problem.
11. Reverse a linked list.
12. Insert in a sorted list
13. In a X’s and 0’s game (i.e. TIC TAC TOE) if you write a program for this give a gast way to generate the moves by the computer. I mean this should be the fasteset way possible. The answer is that you need to store all possible configurations of the board and the move that is associated with that. Then it boils down to just accessing the right element and getting the corresponding move for it. Do some analysis and do some more optimization in storage since otherwise it becomes infeasible to get the required storage in a DOS machine.
14. I was given two lines of assembly code which found the absolute value of a number stored in two’s complement form. I had to recognize what the code was doing. Pretty simple if you know some assembly and some fundaes on number representation.
15. Give a fast way to multiply a number by 7.
16. How would go about finding out where to find a book in a library. (You don’t know how exactly the books are organized beforehand).
17. Linked list manipulation.
18. Tradeoff between time spent in testing a product and getting into the market first.
19. What to test for given that there isn’t enough time to test everything you want to.
20. First some definitions for this problem: a) An ASCII character is one byte long and the most significant bit in the byte is always ‘0′. b) A Kanji character is two bytes long. The only characteristic of a Kanji character is that in its first byte the most significant bit is ‘1′.
Now you are given an array of a characters (both ASCII and Kanji) and, an index into the array. The index points to the start of some character. Now you need to write a function to do a backspace (i.e. delete the character before the given index).
21. Delete an element from a doubly linked list.
22. Write a function to find the depth of a binary tree.
23. Given two strings S1 and S2. Delete from S2 all those characters which occur in S1 also and finally create a clean S2 with the relevant characters deleted.
24. Assuming that locks are the only reason due to which deadlocks can occur in a system. What would be a foolproof method of avoiding deadlocks in the system.
25. Reverse a linked list.
Ans: Possible answers -
iterative loop
curr->next = prev;
prev = curr;
curr = next;
next = curr->next
endloop
recursive reverse(ptr)
if (ptr->next == NULL)
return ptr;
temp = reverse(ptr->next);
temp->next = ptr;
return ptr;
end
26. Write a small lexical analyzer - interviewer gave tokens. expressions like "a*b" etc.
27. Besides communication cost, what is the other source of inefficiency in RPC? (answer : context switches, excessive buffer copying). How can you optimise the communication? (ans : communicate through shared memory on same machine, bypassing the kernel _ A Univ. of Wash. thesis)
28. Write a routine that prints out a 2-D array in spiral order!
29. How is the readers-writers problem solved? - using semaphores/ada .. etc.
30. Ways of optimizing symbol table storage in compilers.
31. A walk-through through the symbol table functions, lookup() implementation etc - The interv. was on the Microsoft C team.
32. A version of the "There are three persons X Y Z, one of which always lies".. etc..
33. There are 3 ants at 3 corners of a triangle, they randomly start moving towards another corner.. what is the probability that they don’t collide.
34. Write an efficient algo and C code to shuffle a pack of cards.. this one was a feedback process until we came up with one with no extra storage.
35. The if (x == 0) y = 0 etc..
36. Some more bitwise optimization at assembly level
37. Some general questions on Lex, Yacc etc.
38. Given an array t[100] which contains numbers between 1..99. Return the duplicated value. Try both O(n) and O(n-square).
39. Given an array of characters. How would you reverse it. ? How would you reverse it without using indexing in the array.
40. GIven a sequence of characters. How will you convert the lower case characters to upper case characters. ( Try using bit vector - sol given in the C lib -typec.h)
41. Fundas of RPC.
42. Given a linked list which is sorted. How will u insert in sorted way.
43. Given a linked list How will you reverse it.
44. Give a good data structure for having n queues ( n not fixed) in a finite memory segment. You can have some data-structure separate for each queue. Try to use at least 90% of the memory space.
45. Do a breadth first traversal of a tree.
46. Write code for reversing a linked list.
47. Write, efficient code for extracting unique elements from a sorted list of array. e.g. (1, 1, 3, 3, 3, 5, 5, 5, 9, 9, 9, 9) -> (1, 3, 5, 9).
48. Given an array of integers, find the contiguous subarray with the largest sum.
ANS. Can be done in O(n) time and O(1) extra space. Scan array from 1 to n. Remember the best subarray seen so far and the best subarray ending in i.
49. Given an array of length N containing integers between 1 and N, determine if it contains any duplicates.
ANS. [Is there an O(n) time solution that uses only O(1) extra space and does not destroy the original array?]
50. Sort an array of size n containing integers between 1 and K, given a temporary scratch integer array of size K.
ANS. Compute cumulative counts of integers in the auxiliary array. Now scan the original array, rotating cycles! [Can someone word this more nicely?]
* 51. An array of size k contains integers between 1 and n. You are given an additional scratch array of size n. Compress the original array by removing duplicates in it. What if k <<>
ANS. Can be done in O(k) time i.e. without initializing the auxiliary array!
52. An array of integers. The sum of the array is known not to overflow an integer. Compute the sum. What if we know that integers are in 2’s complement form?
ANS. If numbers are in 2’s complement, an ordinary looking loop like for(i=total=0;i 53. An array of characters. Reverse the order of words in it.
ANS. Write a routine to reverse a character array. Now call it for the given array and for each word in it.
* 54. An array of integers of size n. Generate a random permutation of the array, given a function rand_n() that returns an integer between 1 and n, both inclusive, with equal probability. What is the expected time of your algorithm?
ANS. "Expected time" should ring a bell. To compute a random permutation, use the standard algo of scanning array from n downto 1, swapping i-th element with a uniformly random element <= i-th. To compute a unformly random integer between 1 and k (k <>
55. An array of pointers to (very long) strings. Find pointers to the (lexicographically) smallest and largest strings.
ANS. Scan array in pairs. Remember largest-so-far and smallest-so-far. Compare the larger of the two strings in the current pair with largest-so-far to update it. And the smaller of the current pair with the smallest-so-far to update it. For a total of <= 3n/2 strcmp() calls. That’s also the lower bound.
56. Write a program to remove duplicates from a sorted array.
ANS. int remove_duplicates(int * p, int size)
{
int current, insert = 1;
for (current=1; current <>
return insert;
}
57. C++ ( what is virtual function ? what happens if an error occurs in constructor or destructor. Discussion on error handling, templates, unique features of C++. What is different in C++, ( compare with unix).
58. Given a list of numbers ( fixed list) Now given any other list, how can you efficiently find out if there is any element in the second list that is an element of the first list (fixed list).
59. GIven 3 lines of assembly code : find it is doing. IT was to find absolute value.
60. If you are on a boat and you throw out a suitcase, Will the level of water increase.
61. Print an integer using only putchar. Try doing it without using extra storage.
62. Write C code for (a) deleting an element from a linked list (b) traversing a linked list
63. What are various problems unique to distributed databases
64. Declare a void pointer ANS. void *ptr;
65. Make the pointer aligned to a 4 byte boundary in a efficient manner ANS. Assign the pointer to a long number and the number with 11…1100 add 4 to the number
66. What is a far pointer (in DOS)
67. What is a balanced tree
68. Given a linked list with the following property node2 is left child of node1, if node2 <>
O P
|
|
O A
|
|
O B
|
|
O C
How do you convert the above linked list to the form without disturbing the property. Write C code for that.
O P
|
|
O B
/ \
/ \
/ \
O ? O ?
determine where do A and C go
69. Describe the file system layout in the UNIX OS
ANS. describe boot block, super block, inodes and data layout
70. In UNIX, are the files allocated contiguous blocks of data
ANS. no, they might be fragmented
How is the fragmented data kept track of
ANS. Describe the direct blocks and indirect blocks in UNIX file system
71. Write an efficient C code for ‘tr’ program. ‘tr’ has two command line arguments. They both are strings of same length. tr reads an input file, replaces each character in the first string with the corresponding character in the second string. eg. ‘tr abc xyz’ replaces all ‘a’s by ‘x’s, ‘b’s by ‘y’s and so on. ANS.
a) have an array of length 26.
put ‘x’ in array element corr to ‘a’
put ‘y’ in array element corr to ‘b’
put ‘z’ in array element corr to ‘c’
put ‘d’ in array element corr to ‘d’
put ‘e’ in array element corr to ‘e’
and so on.
the code
while (!eof)
{
c = getc();
putc(array[c - ‘a’]);
}
72. what is disk interleaving
73. why is disk interleaving adopted
74. given a new disk, how do you determine which interleaving is the best a) give 1000 read operations with each kind of interleaving determine the best interleaving from the statistics
75. draw the graph with performace on one axis and ‘n’ on another, where ‘n’ in the ‘n’ in n-way disk interleaving. (a tricky question, should be answered carefully)
76. I was a c++ code and was asked to find out the bug in that. The bug was that he declared an object locally in a function and tried to return the pointer to that object. Since the object is local to the function, it no more exists after returning from the function. The pointer, therefore, is invalid outside.
77. A real life problem - A square picture is cut into 16 sqaures and they are shuffled. Write a program to rearrange the 16 squares to get the original big square.
78.
int *a;
char *c;
*(a) = 20;
*c = *a;
printf("%c",*c);
what is the output?
79. Write a program to find whether a given m/c is big-endian or little-endian!
80. What is a volatile variable?
81. What is the scope of a static function in C ?
82. What is the difference between "malloc" and "calloc"?
83. struct n { int data; struct n* next}node;
node *c,*t;
c->data = 10;
t->next = null;
*c = *t;
what is the effect of the last statement?
1. Given a rectangular (cuboidal for the puritans) cake with a rectangular piece removed (any size or orientation), how would you cut the remainder of the cake into two equal halves with one straight cut of a knife?
ANS. Join the centers of the original and the removed rectangle. It works for cuboids too!
2. There are 3 baskets. one of them have apples, one has oranges only and the other has mixture of apples and oranges. The labels on their baskets always lie. (i.e. if the label says oranges, you are sure that it doesn’t have oranges only,it could be a mixture) The task is to pick one basket and pick only one fruit from it and then correctly label all the three baskets.
HINT. There are only two combinations of distributions in which ALL the baskets have wrong labels. By picking a fruit from the one labeled MIXTURE, it is possible to tell what the other two baskets have.
3. You have 8 balls. One of them is defective and weighs less than others. You have a balance to measure balls against each other. In 2 weighings how do you find the defective one?
Answer from Uday Venkat: weigh three balls against another three balls. if both weigh the same , then just weighing the remain two (one against one) will show the lighter ball. if the sets of three do not weigh equal, then weigh any two balls in the lighter set, one against the other . the balance will show if the lighter one is on the balance,if not the remaining one is the lighter one.
8= (3 + 3 ) + 2
(the numbers in the brackets are balls on either side of the balance)
if both are equal, then
2= (1 + 1) done.
else, from the lighter set of 3
3= (1 + 1) + 1 done.
4. Why is a manhole cover round?
HINT. The diagonal of a square hole is larger than the side of a cover!
Alternate answers: 1. Round covers can be transported by one person, because they can be rolled on their edge. 2. A round cover doesn’t need to be rotated to fit over a hole.
Here's a list of the most common mistakes made at group discussions:
Emotional outburst
Rashmi was offended when one of the male participants in a group discussion made a statement on women generally being submissive while explaining his point of view. When Rashmi finally got an opportunity to speak, instead of focusing on the topic, she vented her anger by accusing the other candidate for being a male chauvinist and went on to defend women in general.
What Rashmi essentially did was to
• Deviate from the subject
• Treat the discussion as a forum to air her own views.
• Lose objectivity and make personal attacks.
Her behaviour would have been perceived as immature and demotivating to the rest of the team.
Quality Vs Quantity
Gautam believed that the more he talked, the more likely he was to get through the GD. So, he interrupted other people at every opportunity. He did this so often that the other candidates got together to prevent him from participating in the rest of the discussion.
• Assessment is not only on your communication skills but also on your ability to be a team player.
• Evaluation is based on quality, and not on quantity. Your contribution must be relevant.
• The mantra is "Contributing meaningfully to the team's success." Domination is frowned upon.
Egotism Showing off
Krishna was happy to have got a group discussion topic he had prepared for. So, he took pains to project his vast knowledge of the topic. Every other sentence of his contained statistical data - "20% of companies; 24.27% of parliamentarians felt that; I recently read in a Jupiter Report that..." and so on so forth. Soon, the rest of the team either laughed at him or ignored his attempts to enlighten them as they perceived that he was cooking up the data.
• Exercise restraint in anything. You will end up being frowned upon if you attempt showing-off your knowledge.
• Facts and figures need not validate all your statements.
• Its your analysis and interpretation that are equally important - not just facts and figures.
• You might be appreciated for your in-depth knowledge. But you will fail miserably in your people skills.
Such a behavior indicates how self-centered you are and highlights your inability to work in an atmosphere where different opinions are expressed.
Get noticed - But for the right reasons
Srikumar knew that everyone would compete to initiate the discussion. So as soon as the topic - "Discuss the negative effects of India joining the WTO" - was read out, he began talking. In his anxiety to be the first to start speaking, he did not hear the word "negative" in the topic. He began discussing the ways in which the country had benefited by joining WTO, only to be stopped by the evaluator, who then corrected his mistake.
• False starts are extremely expensive. They cost you your admission. It is very important to listen and understand the topic before you air your opinions.
• Spending a little time analyzing the topic may provide you with insights which others may not have thought about. Use a pen and paper to jot down your ideas.
• Listen! It gives you the time to conceptualize and present the information in a better manner.
Some mistakes are irreparable. Starting off the group discussion with a mistake is one such mistake, unless you have a great sense of humor.
Managing one's insecurities
Sumati was very nervous. She thought that some of the other candidates were exceptionally good. Thanks to her insecurity, she contributed little to the discussion. Even when she was asked to comment on a particular point, she preferred to remain silent.
• Your personality is also being evaluated. Your verbal and non verbal cues are being read.
• Remember, you are the participant in the GD; not the evaluator. So, rather than evaluating others and your performance, participate in the discussion.
• Your confidence level is being evaluated. Decent communication skills with good confidence is a must to crack the GDs.
Focus on your strengths and do not spend too much time thinking about how others are superior or inferior to you. It is easy to pick up these cues from your body language.
Knowledge is strength. A candidate with good reading habits has more chances of success. In other words, sound knowledge on different topics like politics, finance, economy, science and technology is helpful.
Power to convince effectively is another quality that makes you stand out among others.
Clarity in speech and expression is yet another essential quality.
If you are not sure about the topic of discussion, it is better not to initiate. Lack of knowledge or wrong approach creates a bad impression. Instead, you might adopt the wait and watch attitude. Listen attentively to others, may be you would be able to come up with a point or two later.
A GD is a formal occasion where slang is to avoided.
A GD is not a debating stage. Participants should confine themselves to expressing their viewpoints. In the second part of the discussion candidates can exercise their choice in agreeing, disagreeing or remaining neutral.
Language use should be simple, direct and straight forward.
Don't interrupt a speaker when the session is on. Try to score by increasing your size, not by cutting others short.
Maintain rapport with fellow participants. Eye contact plays a major role. Non-verbal gestures, such as listening intently or nodding while appreciating someone's viewpoint speak of you positively.
Communicate with each and every candidate present. While speaking don't keep looking at a single member. Address the entire group in such a way that everyone feels you are speaking to him or her.What is IQ?
A General Intelligence Quotient Score (IQ Score) is a statistically derived number which indicates relative and comparative abilities that can be used to obtain academic skills and knowledge.
Some of these measurements can be reliable predictors of an individual's academic achievements.
Though an IQ test measures only a few of a humans mental abilities, these few abilities are targeted for measurement, because they are well known to positively correlate highly to many other human abilities. How high you score in one of these measured abilities, will strongly indicate how high you would be expected to score on the unmeasured abilities. So here are some tips to Improve Your IQ....
Brain exercises.
Math is a great exercise for your brain. When you see an object, think of something it can be used for, apart from what it is usually used for. If you regularly exercise your brain you develop your neurons and can stop or delay the process of mental deterioration that age causes.
Read faster.
Speed reading increases you ability to understand. But many people believe it doesn’t help. Speed reading helps you learn faster and more. A tip: when you scan always read the first and last stanzas because all the crux lies in these contents. Pick up any article and test this tip.
Work out
Exercising regularly enhances the power of your brain. Any regime that is good for your physical fitness boosts brain power too. Latest research has shown that an exercise as short as a 10 minute aerobics is sufficient to activate your brain. Hence, if you want to freshen your brain, just climb up and down your stairs a few times.
Rosemary
Many people claim that this herb has effect on the brain. No research has been done though. Just sniffing seems to stimulate your brain. It is a very safe technique, no side effects hence no harm in trying it out.
Smart learning
Always make it a habit when you learn something new. Always have some question to ask for each session of learning that you take. Take short breaks, because things at the starting and ending of a class last in your memory for a longer time.
Have conducive surroundings
Congested places and surroundings can affect your thought process. Have a conducive environment to do any mental task. Simple techniques like deep breathing, stretching can help you gear up before you begin the mental task. Always have time to divert your mind.
Creatine
Creatine, is found in meat and is used by sportspersons to help become muscular. Research has shown results which say that creatine also is beneficial to the brain. Proceedings B, a Royal Society journal has published the results. These results say that a remarkable improvement in the working memory and intelligence level was observed when a person takes creatine. The recommended dosage is 5 grams in a day. This is what most sportspersons take and can be found in 4 pounds of meet.
Talking
Talking, if done judiciously, will help you clear your understanding about things. Try to explain a concept about which you yourself do not have a clear idea. You will notice that this clears up your understanding to a large extent.
Use brain wave tools
This is the latest tool. These brain wave entertainment tools help you a lot. They can relax you quickly and some of them can make your brain start analyzing things in a different manner. You can try BrainWave stimulator software.
Indulge in what you love to do
This reduces stress and rejuvenates your brain. But it should be not something like watching TV. It should be an activity in which your are fully involved like playing scrabble or other similar games. When you indulge in what you love to do, you tend to worry less and this clears up your thought process.
Be positive
Be confident about yourself and you will become smart. Affirmations will be of great help. Always remember things you succeeded in. Note down all the creative ideas that come up in your brain. Note all things you do in a smart ways and you will grow smarter.
Follow creative people’s methods.
Always be in the company of those who are smart and productive. Observe them and do what they do. This is the basic principle behind neuron linguistic programming. But be cautious, when anyone of them advise you. Usually successful people don’t fully understand the reason of their success. Remember to follow what they do, not what they say!
Avoid getting into debates
Arguments always arouse your ego. When you are defending your view, you bring ego and not logic. This adversely affects your brain. The more you argue, the more you destabilize your brain. Arguments and debates can be value adding, but only if ego is not involved. Once ego enters, all logic fails. This certainly doesn’t help your IQ.
Avoid allergic food items.
Some food items like wheat, corn, peanuts and diary products may cause some minor allergies. Be observant to notice such effects. Some people face digestion problems and even brain fog by eating such items.
Eat fish.
It has been proven that consuming fish speeds up your brain and helps you focus for longer durations. Research has depicted a strong relation between lowering of depression and fish consumption. For instance, Japan (where fish is the staple food) has far less instances of depression than US.
Networking- is the practice of linking computing devices together with hardware and software that supports data communications across these devices.
some frequently asked Questions...
1. What are 10Base2, 10Base5 and 10BaseT Ethernet LAN?
2. What are the two types of transmission technology available?
3. What is point-to-point protocol?
5. What are the possible ways of data exchange?
6. What is difference between baseband and broadband transmission?
7. What is Protocol Data Unit?
8. What are major types of networks and explain?
9. What are the important topologies for networks?
10. What is passive topology?
11. What is mesh network?
12. What are the different type of networking / internet working devices?
13. How Gateway is different from Routers?
14. What is router?
15. What is subnet?
16. What is SAP?
17. What do you meant by "triple X" in Networks?
18. What is frame relay, in which layer it comes?
19. What is terminal emulation, in which layer it comes?
20. What is Beaconing?
21. What is redirector?
22. What is NETBIOS and NETBEUI?
23. What is cladding?
24. What is attenuation?
25. What is MAC address?
26. Difference between bit rate and baud rate.Bit rate is the number of bits transmitted during one sec?
27. What is Bandwidth?
28. What is Project 802?
29. What are the data units at different layers of the TCP / IP protocol suite?
30. What is ICMP?
31. What is difference between ARP and RARP?
32. What is the range of addresses in the classes of internet addresses?
33. Why should you care about the OSI Reference Model?
34. What is the difference between routable and non- routable protocols?
35. What is multicast routing?
Snow Effect

Contact


Contributors
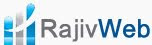
- Rajiv Pandey
- Visit here to know more about me - http://www.rajivweb.com/about-me/
Recent Posts
Blog Archive
- September 2012 (1)
- February 2009 (3)
- January 2009 (3)
- December 2008 (4)
- November 2008 (2)
- October 2008 (2)
- September 2008 (3)
- August 2008 (4)
- July 2008 (3)
- June 2008 (5)
- May 2008 (2)
- April 2008 (3)
- March 2008 (1)
- February 2008 (3)
- January 2008 (2)
- December 2007 (5)
- November 2007 (6)
- October 2007 (8)
Categories
- Bidding Directory
- Business
- Career
- Creative
- Data Flow
- Deep Bidding Directory
- Free Online Games
- Future of IT
- Improve
- Internet
- Interview Tips
- Java
- Keyword Density
- Knowledge
- law
- Link
- Link Building
- List of Blogs
- Meta
- Meta Tags
- News
- On-page optimization
- Page Rank
- Question Papers
- Search Engine
- SEM
- SEO
- Tips
- Web2.0
- Website